OpenAI의 Batch API는 동시 다발적인 요청을 처리하면서도 50% 더 저렴한 비용으로 대규모 데이터를 처리할 수 있는 효과적인 도구이다. 즉각적인 응답이 필요하지 않거나 대규모 데이터를 효율적으로 처리하고 싶은 경우에 유용하며, 특히 큰 데이터셋을 분석하거나 대량의 작업을 한 번에 처리하는 데 적합하다. 이번 포스트에서는 Batch API 사용법을 초보자들도 쉽게 따라할 수 있도록 설명하고 예제를 포함하여 안내해 보고자 한다.
Batch API란?
Batch API는 OpenAI 플랫폼에서 제공하는 비동기식 요청 처리를 위한 API이다. Batch API를 사용하면 다음과 같은 이점이 있다.
- 비용 절감: 동기식 API 대비 50% 저렴한 비용으로 처리 가능.
- 높은 처리 한도: 동기식 API에 비해 더 많은 요청을 처리할 수 있는 별도의 처리 한도를 제공.
- 빠른 처리 시간: 각 배치는 24시간 이내에 완료되며, 대부분의 경우 더 빠르게 완료된다.
주로 사용하는 용도로는 다음과 같은 작업들이 있다
- 대량 데이터 분류
- 대규모 콘텐츠 임베딩
- 다양한 작업에 대한 평가 수행
시작하기
1단계: Batch 파일 준비하기
먼저, 처리할 요청들을 하나의 .jsonl
파일에 담아야 한다. 파일의 각 줄에는 하나의 API 요청이 포함되며, 각각 고유한 custom_id
값을 가져야 한다. 아래는 두 개의 요청을 담은 예시이다.
{"custom_id": "request-1", "method": "POST", "url": "/v1/chat/completions", "body": {"model": "gpt-3.5-turbo", "messages": [{"role": "system", "content": "You are a helpful assistant."}, {"role": "user", "content": "Hello world!"}], "max_tokens": 1000}} {"custom_id": "request-2", "method": "POST", "url": "/v1/chat/completions", "body": {"model": "gpt-3.5-turbo", "messages": [{"role": "system", "content": "You are an unhelpful assistant."}, {"role": "user", "content": "Hello world!"}], "max_tokens": 1000}}
이 .jsonl
파일을 기반으로 여러 요청을 한 번에 처리할 수 있다. 각 요청은 고유한 custom_id
로 구분되므로 결과를 쉽게 매칭할 수 있다.
2단계: Batch 파일 업로드하기
이제 준비한 Batch 파일을 OpenAI API에 업로드해야 한다. 이를 위해 Files API
를 사용한다.
from openai import OpenAI import os from decouple import config api_key = config('OPENAI_API_KEY') client = OpenAI(api_key=api_key) batch_input_file = client.files.create( file=open("batchinput.jsonl", "rb"), purpose="batch" )
업로드가 성공하면, 해당 파일의 ID를 가져와 Batch 처리를 시작할 수 있다.
3단계: Batch 생성하기
업로드한 파일의 ID를 이용해 Batch를 생성한다. Batch가 생성되면 OpenAI 서버에서 해당 작업을 처리한다.
batch_input_file_id = batch_input_file.id client.batches.create( input_file_id=batch_input_file_id, endpoint="/v1/chat/completions", completion_window="24h", metadata={"description": "nightly eval job"})
4단계: Batch 상태 확인하기
Batch 처리가 진행되는 동안, 언제든지 상태를 확인할 수 있다. Batch 상태는 validating
, in_progress
, completed
등 여러 상태 중 하나일 수 있다.
client.batches.retrieve("batch_id")
5단계: Batch 결과 가져오기
Batch 처리가 완료되면, 결과 파일을 다운로드할 수 있다. 이를 위해 output_file_id
를 사용해 결과를 요청한다.
file_response = client.files.content("output_file_id") print(file_response.text)
Batch API 실습 예제
이제 실제 예제를 통해 Batch API를 더 자세히 살펴보자.
예를 들어, 간단한 단답형 질문 두 개의 요청을 담은 batchinput.jsonl
파일을 생성하고 이를 처리해 보겠다.
1단계: Batch 파일 생성
{"custom_id": "request-1", "method": "POST", "url": "/v1/chat/completions", "body": {"model": "gpt-3.5-turbo", "messages": [{"role": "system", "content": "You are a helpful assistant."}, {"role": "user", "content": "What is the capital of France?"}], "max_tokens": 50}} {"custom_id": "request-2", "method": "POST", "url": "/v1/chat/completions", "body": {"model": "gpt-3.5-turbo", "messages": [{"role": "system", "content": "You are a helpful assistant."}, {"role": "user", "content": "What is the square root of 16?"}], "max_tokens": 50}}
이 파일을 batchinput.jsonl
로 저장한 뒤 업로드한다.
2단계: 파일 업로드 및 Batch 생성
from openai import OpenAI import os from decouple import config api_key = config('OPENAI_API_KEY') client = OpenAI(api_key=api_key) batch_input_file = client.files.create( file=open("batchinput.jsonl", "rb"), purpose="batch" ) batch_input_file_id = batch_input_file.id client.batches.create( input_file_id=batch_input_file_id, endpoint="/v1/chat/completions", completion_window="24h", metadata={"description": "Simple batch job"} )
위와 같이 실행하였다면 다음과 같이 batch id
를 포함한 출력을 받을 수 있다.
Batch(id='batch_66fac1b74b4c81909e46ed0dbebb59a5', completion_window='24h', created_at=1727709623, endpoint='/v1/chat/completions', input_file_id='file-E59g7PZLwXqxXGheg2mwCcLt', object='batch', status='validating', cancelled_at=None, cancelling_at=None, completed_at=None, error_file_id=None, errors=None, expired_at=None, expires_at=1727796023, failed_at=None, finalizing_at=None, in_progress_at=None, metadata={'description': 'nightly eval job'}, output_file_id=None, request_counts=BatchRequestCounts(completed=0, failed=0, total=0))
3단계: Batch 상태 확인 및 결과 확인
Batch 상태를 확인하기
# Batch 상태 확인 client.batches.retrieve("batch_66fac1b74b4c81909e46ed0dbebb59a5")
위와 같은 코드를 실행하면, Batch API가 실행이 진행되거나, 완료 된 뒤 아래와 같이 완료되었다는 status와 output_file_id를 포함한 출력을 받을 수 있다.
Batch(id='batch_66fac1b74b4c81909e46ed0dbebb59a5', completion_window='24h', created_at=1727710409, endpoint='/v1/chat/completions', input_file_id='file-vsSDhngHCO7373T4PYWufBT6', object='batch', status='finalizing', cancelled_at=None, cancelling_at=None, completed_at=None, error_file_id=None, errors=None, expired_at=None, expires_at=1727796809, failed_at=None, finalizing_at=1727710427, in_progress_at=1727710410, metadata={'description': 'Simple batch job'}, output_file_id=None, request_counts=BatchRequestCounts(completed=2, failed=0, total=2))
Batch(id='batch_66fac1b74b4c81909e46ed0dbebb59a5', completion_window='24h', created_at=1727709623, endpoint='/v1/chat/completions', input_file_id='file-E59g7PZLwXqxXGheg2mwCcLt', object='batch', status='completed', cancelled_at=None, cancelling_at=None, completed_at=1727709719, error_file_id=None, errors=None, expired_at=None, expires_at=1727796023, failed_at=None, finalizing_at=1727709718, in_progress_at=1727709624, metadata={'description': 'nightly eval job'}, output_file_id='file-hupoxAnlJc7ao40CIG0WCXsC', request_counts=BatchRequestCounts(completed=2, failed=0, total=2))
OpenAI의 Dashboard에서도 실행중인 Batch들과 진행상황을 모니터링 할 수 있다.
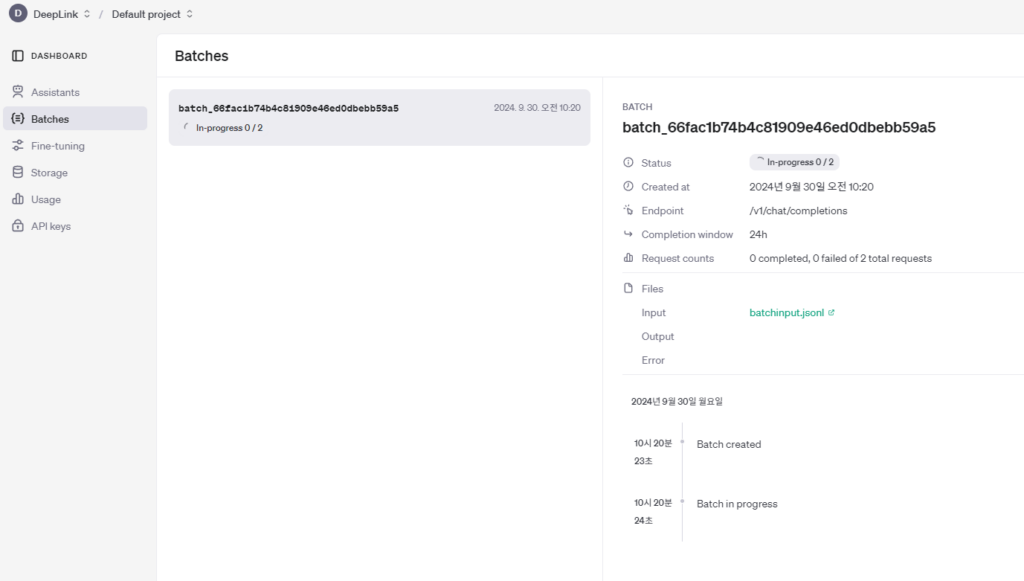
Batch 결과 확인하기
# 결과 파일 가져오기 file_response = client.files.content("file-hupoxAnlJc7ao40CIG0WCXsC") print(file_response.text)
결과 파일에는 각 요청에 대한 응답이 다음과 같이 포함되며, 실패한 요청에 대한 오류 정보는 별도의 파일로 제공된다.
{"id": "batch_req_66fac216857881909c0ff1f5e3a94b39", "custom_id": "request-1", "response": {"status_code": 200, "request_id": "12ea0ae56cf6cb5049286bf77f3eb7bf", "body": {"id": "chatcmpl-ADCVSC4CKycs3BfEkIEwfyc8Xdu17", "object": "chat.completion", "created": 1727709702, "model": "gpt-3.5-turbo-0125", "choices": [{"index": 0, "message": {"role": "assistant", "content": "The capital of France is Paris.", "refusal": null}, "logprobs": null, "finish_reason": "stop"}], "usage": {"prompt_tokens": 24, "completion_tokens": 7, "total_tokens": 31, "completion_tokens_details": {"reasoning_tokens": 0}}, "system_fingerprint": null}}, "error": null} {"id": "batch_req_66fac21695f08190aeabb5dcf222a045", "custom_id": "request-2", "response": {"status_code": 200, "request_id": "ee076077ab41faccc5f10bc3fd73b390", "body": {"id": "chatcmpl-ADCVXmJe7EZOgZ2emcROQaIg29k4p", "object": "chat.completion", "created": 1727709707, "model": "gpt-3.5-turbo-0125", "choices": [{"index": 0, "message": {"role": "assistant", "content": "The square root of 16 is 4.", "refusal": null}, "logprobs": null, "finish_reason": "stop"}], "usage": {"prompt_tokens": 26, "completion_tokens": 10, "total_tokens": 36, "completion_tokens_details": {"reasoning_tokens": 0}}, "system_fingerprint": null}}, "error": null}
Batch API 활용 시 주의사항
- 요청 수 제한: Batch API는 한 번에 최대 50,000개의 요청을 포함할 수 있으며, 입력 파일 크기는 최대 100MB이다.
- 응답 시간: Batch API는 24시간 이내에 처리 완료되며, 대부분의 경우 더 빠르게 완료된다.
- 토큰 제한: Batch API는 별도의 토큰 처리 한도를 가지며, 이를 활용해 기존 동기식 API의 토큰 한도를 넘어선 대규모 처리를 수행할 수 있다.
결론
Batch API는 대량의 데이터를 저렴하고 효율적으로 처리할 수 있는 매우 유용한 도구이다. 특히, 즉각적인 응답이 필요하지 않은 대규모 작업에 적합하며, 비용 절감과 높은 처리 한도를 제공한다. 이 포스트를 통해 Batch API 사용법을 익히고, 실제로 프로젝트에 적용해보자!