In data science and machine learning, dealing with high-dimensional data is crucial for problem-solving. However, as the dimensionality increases, the computational cost rises, and the risk of overfitting grows. Principal Component Analysis (PCA) is a widely used technique to tackle these issues by reducing the dimensionality of the data. In this post, we will explore what PCA is, how it works, and how to implement it in Python.
What is Principal Component Analysis (PCA)?
Principal Component Analysis (PCA) is an algorithm that transforms high-dimensional data into a lower-dimensional space while preserving as much important information as possible. It identifies new axes (principal components) that maximize the variance in the data and projects the data onto these axes.
Main purposes of PCA
- Dimensionality reduction: Reduces the complexity of the data, improving model efficiency.
- Data visualization: Transforms data into 2D or 3D for easier visualization.
- Noise reduction: Removes irrelevant information, enhancing analysis performance.
How PCA Works
PCA involves the following steps to reduce data dimensions:
- Data normalization: Normalize the data so that each variable has a mean of 0 and a variance of 1.
- Compute the covariance matrix: Calculate the covariance matrix to understand the variance relationships between variables.
- Eigenvalue decomposition: Decompose the covariance matrix to find eigenvalues and eigenvectors.
- Select principal components: Choose the eigenvectors corresponding to the largest eigenvalues as the principal components.
- Transform the data: Project the data onto the selected principal components to reduce its dimensions.
Python Code Implementation
Below is a Python code snippet to implement PCA:
import numpy as np
import matplotlib.pyplot as plt
from sklearn.decomposition import PCA
# Generate example data
X1 = np.random.multivariate_normal([3, 5, 2], [[0.1, 0, 0], [0, 0.1, 0], [0, 0, 0.1]], 50)
X2 = np.random.multivariate_normal([6, 2, 6], [[0.1, 0, 0], [0, 0.1, 0], [0, 0, 0.1]], 50)
X3 = np.random.multivariate_normal([7, 2, 7], [[0.1, 0, 0], [0, 0.1, 0], [0, 0, 0.1]], 50)
X = np.vstack((X1, X2, X3))
y = np.array([0] * 50 + [1] * 50 + [2] * 50)
# 예제 데이터 시각화
fig = plt.figure(figsize=(12, 6))
ax = fig.add_subplot(121, projection='3d')
sc = ax.scatter(X[:, 0], X[:, 1], X[:, 2], c=y, cmap='viridis', edgecolor='k')
ax.set_title('Original 3D Data')
ax.set_xlabel('Feature 1')
ax.set_ylabel('Feature 2')
ax.set_zlabel('Feature 3')
# Apply PCA
pca = PCA(n_components=2) # 2차원으로 축소
X_pca = pca.fit_transform(X)
# Visualize the result
ax2 = fig.add_subplot(122)
scatter = ax2.scatter(X_pca[:, 0], X_pca[:, 1], c=y, cmap='viridis', edgecolor='k')
ax2.set_title('PCA Result (2D Projection)')
ax2.set_xlabel('Principal Component 1')
ax2.set_ylabel('Principal Component 2')
plt.tight_layout()
plt.show()
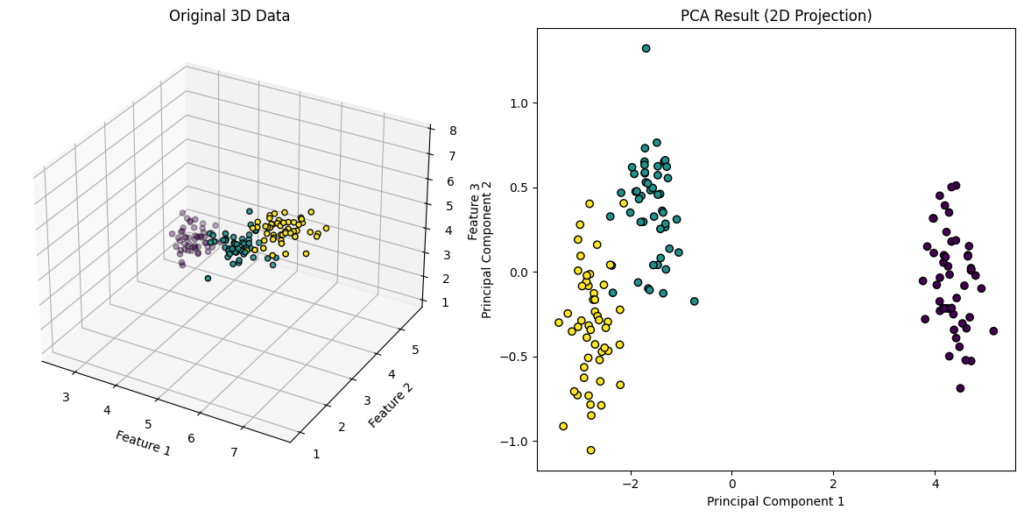
Code Explanation
PCA(n_components=2)
: Reduces the data to 2 dimensions.fit_transform
: Fits the PCA model and transforms the data.plt.scatter
: Visualizes the 2D reduced data.
Advantages and Disadvantages of PCA
Advantages:
- Preserves essential information in the data
- Reduces computational cost by lowering the dimensionality
- Facilitates understanding of data structure through visualization
Disadvantages:
- Limited to linear transformations, which may be inadequate for non-linear data
- Principal components may be difficult to interpret as they may not directly relate to original features
Conclusion
Principal Component Analysis (PCA) is a powerful tool for data reduction and visualization. By using PCA, you can maintain essential information while simplifying data complexity, improving analysis and modeling efficiency. Try implementing the provided code and applying it to different datasets to see PCA in action. It is a fundamental skill for any data scientist due to its simplicity and effectiveness.