Depth-First Search (DFS) is a widely used search algorithm in graph and tree data structures. It starts from a node and explores as far as possible along each branch before backtracking and moving to another branch.
In this post, we will cover the theoretical concepts behind DFS, explain the data structures involved, and provide a detailed Python implementation.
Theoretical Background of DFS
DFS is a method for visiting nodes in structures like trees or graphs. Starting from a node, it explores as deep as possible along one path, and when no further nodes can be explored, it backtracks to the previous branch to explore other paths.
DFS can be implemented using a stack, and the same result can also be achieved through recursion.
Key Features of DFS
- Order of Visit: DFS follows a path as deep as possible and only backtracks when there are no more nodes to visit along that path.
- Completeness: DFS visits all nodes if the graph is finite.
- Non-linear Structure Exploration: DFS can be used not only in trees but also in graphs with cycles.
- Path Finding: DFS is useful for finding paths between nodes and solving problems like maze exploration.
Data Structures
To implement DFS, it’s important to understand the following data structures:
Graph
A graph consists of nodes (vertices) and edges that connect them. In DFS, we visit nodes and edges, and graphs can be represented using adjacency lists or adjacency matrices.
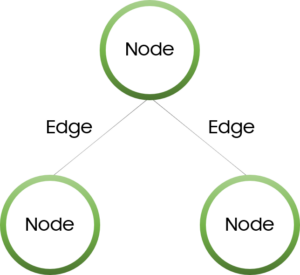
Stack
DFS primarily uses a stack, which follows a Last In, First Out (LIFO) order. The stack helps manage the exploration order in DFS.
Visited Set
To avoid revisiting the same nodes, we need to track whether a node has been visited. This can be done using a set or list.
DFS Algorithm Steps
- Add the starting node to the stack and mark it as visited.
- Pop the top node from the stack and visit it.
- Add all adjacent nodes of the current node to the stack (excluding nodes that have already been visited).
- Repeat this process until the stack is empty.
DFS in Python
Now, let’s look at how DFS can be implemented in Python. We will explore both the recursive and iterative approaches using a stack.
Recursive DFS Implementation
# Graph represented as an adjacency list graph = { 'A': ['B', 'C'], 'B': ['D', 'E'], 'C': ['F'], 'D': [], 'E': ['F'], 'F': [] } # DFS function (recursive implementation) def dfs_recursive(node, visited): if node not in visited: print(node, end=' ') # Print the visited node visited.add(node) # Mark the node as visited for neighbor in graph[node]: # Recursively visit adjacent nodes dfs_recursive(neighbor, visited) # Set to track visited nodes visited = set() # Start DFS dfs_recursive('A', visited)
Code Explanation
- graph: The graph is represented as an adjacency list, where each key is a node, and its value is a list of adjacent nodes.
- dfs_recursive function: This function performs DFS recursively. It marks the current node as visited, then recursively visits adjacent nodes.
- visited: A set to keep track of visited nodes.
Iterative DFS Implementation Using a Stack
# Graph represented as an adjacency list graph = { 'A': ['B', 'C'], 'B': ['D', 'E'], 'C': ['F'], 'D': [], 'E': ['F'], 'F': [] } # DFS function (iterative implementation using a stack) def dfs_stack(start_node): visited = set() # Set to track visited nodes stack = [start_node] # Start with the initial node in the stack while stack: node = stack.pop() # Pop a node from the stack if node not in visited: print(node, end=' ') # Print the visited node visited.add(node) # Mark the node as visited stack.extend(reversed(graph[node])) # Add adjacent nodes to the stack in reverse order for correct traversal # Start DFS dfs_stack('A')
Code Explanation
- dfs_stack function: This function performs DFS iteratively using a stack. It pops nodes from the stack, checks if they’ve been visited, and adds adjacent nodes to the stack.
- stack: The stack holds the nodes to be explored. Here, adjacent nodes are added in reverse order to ensure the correct traversal order.
Use Cases of DFS
DFS is useful in a variety of problems, including:
- Pathfinding: Finding a path between two nodes.
- Maze Solving: Finding an exit in a maze.
- Cycle Detection: Detecting cycles in a graph.
- Topological Sorting: Sorting tasks with a defined order.
Conclusion
In this post, we covered the theoretical background of Depth-First Search (DFS), explained the necessary data structures, and demonstrated how to implement DFS in Python using both recursive and iterative approaches. DFS is an important algorithm for traversing trees and graphs and can be applied to solve a wide range of problems. With the provided example code, I hope you’ve gained a good understanding of DFS and are ready to apply it to algorithmic challenges.