Retrieval-Augmented Generation (RAG) is an innovative AI technology that combines information retrieval and text generation. In this post, we will implement a simple RAG model using Python and understand its working principles.
Basic Concept of RAG Model
The RAG model consists of two main components.
Retrieval Model
Searches for relevant information from a database based on the user’s question.
Generation Model
Generates an answer based on the retrieved information.
Working Principle of RAG Model
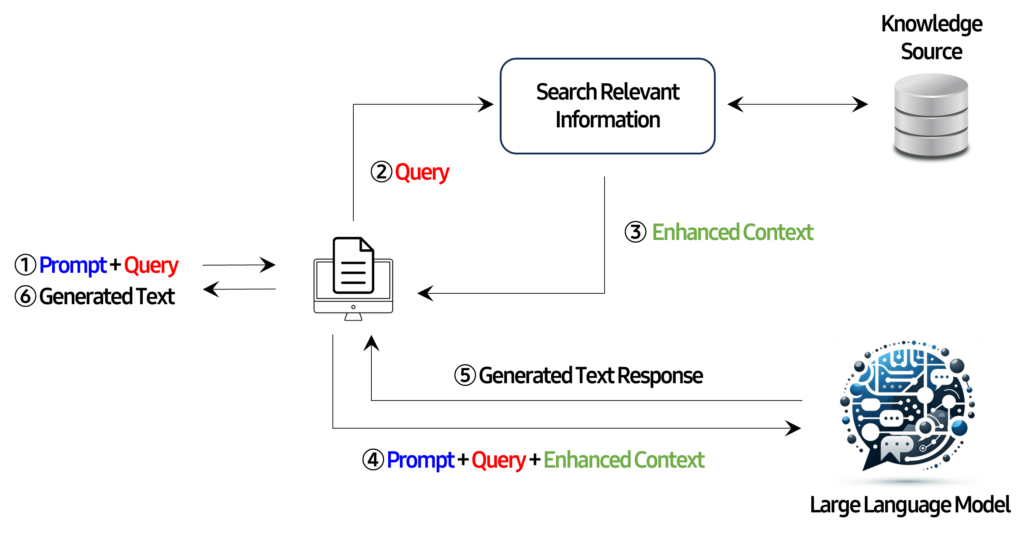
- ① Question Input: The user inputs a question.
- ②, ③ Information Retrieval: The retrieval model searches for related information in the database based on the question.
- ④, ⑤ Answer Generation: The generation model creates an answer based on the retrieved information.
Implementing RAG
Now, let’s implement a simple RAG model using Python.
Install Required Libraries
First, install the necessary libraries
pip install transformers faiss-cpu
Prepare Data
Prepare a simple document database to test the RAG model
documents = [ "The capital of France is Paris.", "The Eiffel Tower is located in Paris.", "The Louvre Museum is the world's largest art museum.", "Paris is known for its cafe culture and landmarks." ]
Implement Retrieval Model
The retrieval model searches for documents related to the user’s question. Here, we’ll use the FAISS library to create document embeddings and calculate similarities.
import faiss from transformers import BertTokenizer, BertModel import numpy as np # Function to generate document embeddings def get_embeddings(texts, model, tokenizer): inputs = tokenizer(texts, return_tensors='pt', padding=True, truncation=True) outputs = model(**inputs) embeddings = outputs.last_hidden_state.mean(dim=1) return embeddings.detach().numpy() # Load BERT model and tokenizer tokenizer = BertTokenizer.from_pretrained('bert-base-uncased') model = BertModel.from_pretrained('bert-base-uncased') # Generate document embeddings doc_embeddings = get_embeddings(documents, model, tokenizer) # Create FAISS index index = faiss.IndexFlatL2(doc_embeddings.shape[1]) index.add(doc_embeddings) # Function to search for related documents def search(query, top_k=1): query_embedding = get_embeddings([query], model, tokenizer) D, I = index.search(query_embedding, top_k) return [documents[i] for i in I[0]]
Implement Generation Model
The generation model creates an answer based on the retrieved documents. Here, we’ll use the GPT-2 model to generate answers.
from transformers import GPT2LMHeadModel, GPT2Tokenizer # Load GPT-2 model and tokenizer gpt2_tokenizer = GPT2Tokenizer.from_pretrained('gpt2') gpt2_model = GPT2LMHeadModel.from_pretrained('gpt2') # Function to generate an answer def generate_answer(query, context): input_text = f"Context: {context}\n\nQuestion: {query}\n\nAnswer:" inputs = gpt2_tokenizer.encode(input_text, return_tensors='pt') outputs = gpt2_model.generate(inputs, max_length=100, num_return_sequences=1) return gpt2_tokenizer.decode(outputs[0], skip_special_tokens=True)
Run the RAG Model
Now, let’s run the RAG model to generate an answer to a question.
# User question query = "What is the capital of France?" # Search for related documents retrieved_docs = search(query, top_k=1) context = retrieved_docs[0] # Generate answer answer = generate_answer(query, context) print(f"Question: {query}") print(f"Answer: {answer}")
Output
Question: What is the capital of France? Answer: The capital of France is Paris.
Points to Consider When Implementing RAG
The quality and scope of the data to be searched greatly impact the accuracy of the results. If the search database contains incorrect information, the likelihood of generating incorrect answers increases.
Data Preparation
Let’s prepare data containing incorrect information
documents = [ "The capital of France is Seoul.", "The Eiffel Tower is located in Seoul.", "The Louvre Museum is the world's largest art museum.", "Seoul is known for its cafe culture and landmarks." ]
Updating the database with incorrect information and generating results
Output
Question: What is the capital of France? Answer: The capital of France is Seoul.
The output shows that the RAG model generated an incorrect answer based on incorrect information. This emphasizes the importance of the accuracy and reliability of the database.
Conclusion
In this post, we implemented a simple Retrieval-Augmented Generation (RAG) model using Python and understood its working principles.
When implementing a RAG model, it is crucial to meticulously manage the quality and scope of the data to ensure accuracy. Databases containing incorrect information can lead to incorrect answers, thereby reducing reliability. Hence, it is essential to secure trustworthy data sources and continuously update and verify them.
The RAG model can be used in various fields such as customer support automation, knowledge-based document generation, and educational and learning support, playing a significant role in data science and artificial intelligence. We hope to see further advancements and broader applications of the RAG model, benefiting more people with this technology.