What is Scikit-Learn?
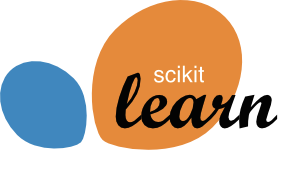
Scikit-Learn is a free software machine learning library for the Python programming language. It provides simple and efficient tools for data mining and data analysis, implementing a wide variety of machine learning algorithms for scientific and engineering purposes.
Scikit-Learn is one of the most popular and widely used machine learning libraries, known for its simplicity and versatility.
Scikit-Learn supports various machine learning tasks, including classification, regression, clustering, dimensionality reduction, model selection, and preprocessing. It offers a simple and consistent API that makes it easy to use and integrate various machine learning algorithms.
Scikit-Learn Key Features
- Classification: Classifies samples into two or more classes. For example, it is used to identify spam emails or recognize characters.
- Regression: Predicts continuous values for data points. For example, it is used to predict housing prices or stock prices.
- Clustering: Groups similar samples to structure the data. For example, it is used for customer segmentation or data summarization.
- Dimensionality Reduction: Reduces the dimensionality of high-dimensional data for visualization or efficient computation.
- Model Selection: Finds the optimal parameters to improve the performance of the model and evaluate its generalization ability.
- Preprocessing: Normalizes data, handles missing values, and extracts or selects features.
Installation of Scikit-Learn
Before installing Scikit-Learn, you need to have Python and dependencies like NumPy and SciPy installed. The easiest way to install Scikit-Learn is by using pip.
- Installation using pip
pip install scikit-learn
This command automatically installs Scikit-Learn and all necessary dependencies. - Installation using Anaconda
If you are using Anaconda, you can install Scikit-Learn using the Conda package manager.conda install scikit-learn
- Installation from source code
If you want to install the latest or development version, you can clone the source code from GitHub and build it yourself.git clone https://github.com/scikit-learn/scikit-learn.git
cd scikit-learn
pip install .
Example of Using Scikit-Learn
Here is an example code using Scikit-Learn to solve a simple classification problem.
from sklearn import datasets from sklearn.model_selection import train_test_split from sklearn.preprocessing import StandardScaler from sklearn.neighbors import KNeighborsClassifier from sklearn.metrics import accuracy_score # Load dataset iris = datasets.load_iris() X = iris.data y = iris.target # Split data X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2) # Preprocess data scaler = StandardScaler() X_train = scaler.fit_transform(X_train) X_test = scaler.transform(X_test) # Train model model = KNeighborsClassifier(n_neighbors=5) model.fit(X_train, y_train) # Predict and evaluate y_pred = model.predict(X_test) accuracy = accuracy_score(y_test, y_pred) print(f'Accuracy: {accuracy:.2f}')
In this example, we load the iris dataset, split the data into training and test sets, and then use the K-Nearest Neighbors classifier to train and evaluate the model.
Scikit-Learn is a valuable tool for data scientists and researchers to develop machine learning models, analyze data, and solve complex data problems.